How to Work with TCP Sockets in Python
Programming and Development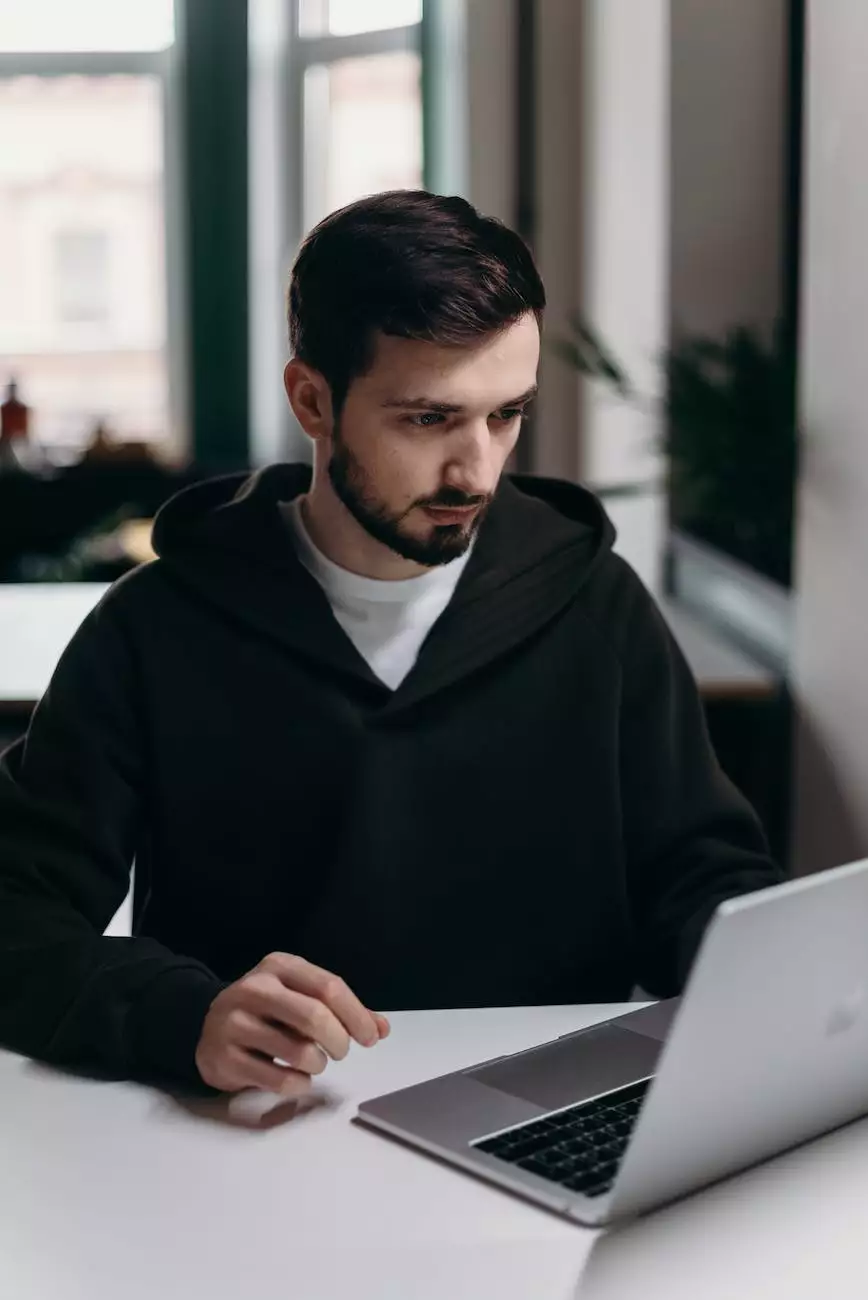
Introduction
Welcome to AwesomeWebsites4Free's comprehensive guide on working with TCP sockets in Python! If you're diving into socket programming or looking to enhance your networking skills, you've come to the right place. This guide will walk you through the essentials of TCP sockets, teach you how to establish connections, send and receive data, and handle errors effectively. Let's get started!
What are TCP Sockets?
TCP (Transmission Control Protocol) sockets are a communication mechanism widely used in computer networks. They allow processes running on different machines to establish reliable connections and exchange data. Python provides a powerful socket module that simplifies the creation and management of TCP sockets.
Setting Up a TCP Socket
To begin working with TCP sockets in Python, you'll first need to import the socket module. This module provides the necessary functions and constants for socket programming. Once imported, you can create a socket object like this:
import socket # Create a TCP socket sock = socket.socket(socket.AF_INET, socket.SOCK_STREAM)Establishing a Connection
Establishing a TCP connection involves two endpoints: a server and a client. The server creates a socket and listens for incoming connections, while the client initiates the connection by specifying the server's IP address and port number. Let's see how this works:
import socket # Server side: server_socket = socket.socket(socket.AF_INET, socket.SOCK_STREAM) server_address = ('localhost', 8000) server_socket.bind(server_address) server_socket.listen(1) # Client side: client_socket = socket.socket(socket.AF_INET, socket.SOCK_STREAM) server_address = ('localhost', 8000) client_socket.connect(server_address)Sending and Receiving Data
Once a connection is established, data can be sent and received between the server and client. The send() and recv() methods facilitate this data exchange. It's important to note that data sent and received over TCP sockets is in the form of bytes.
import socket # Server side: client_socket, client_address = server_socket.accept() data = client_socket.recv(1024) # Client side: message = "Hello, server!" client_socket.send(message.encode()) response = client_socket.recv(1024)Error Handling
Working with TCP sockets entails handling potential errors to ensure smooth communication. Common issues include connection timeouts, broken connections, and errors during data transmission. Utilizing exception handling is crucial to handle these situations gracefully and maintain robust network applications.
Conclusion
Congratulations! You now have a solid understanding of working with TCP sockets in Python. This guide covered the basics of setting up a socket, establishing connections, sending and receiving data, and handling errors efficiently. Utilize this knowledge to create powerful network applications, bolstering your skills in Python and networking in general. Happy coding!
Resources
- Official Python Socket Documentation
- Real Python - Socket Programming
- GeeksforGeeks - Socket Programming in Python
Thank you for choosing AwesomeWebsites4Free as your destination for learning and mastering TCP sockets in Python! Keep exploring our website for more exciting tutorials and resources in the eCommerce & Shopping category.